Display a recommendation title for the zone
You can display a recommendation title for each zone. The recommendation title is specified your product recommendations strategy. Displaying the recommendation title communicates your recommendation strategy goal to your website visitors.
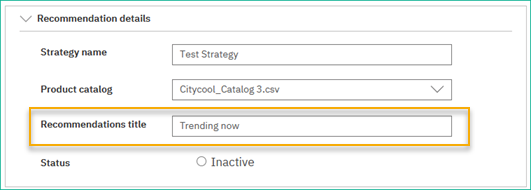
Recommendations titles are optional. You can leave the field empty. You can also update the recommendation titles manually on your website. To display the recommendation title, you must change your zone configuration code.
Recommendation title for MPA channel zone
Deploy Personalization library version 2.2.0 on your MPA channel.
Zone configuration
- Configure MPA zone for product recommendations. For more information, see Configure zones on your channel.
- In the
"recommendation"
section, add the following code.
var personalizationHandler = function(result, targetArea, headerElement)
{
switch(result.type) {
//Use to display product recommendations on the zone
case "recommendation":
displayRecommendations(result, targetArea, headerElement);
break;
. . .
. . .
}
- In the helper functions in
personalizationHandler()
function, update thedisplayRecommendation()
function as shown:
var displayRecommendations = function (products, targetArea, headerElementId) {
var recommendations = products.value;
if (products.header && headerElementId) {
document.getElementById(headerElementId).innerHTML = products.header;
}
if (recommendations && recommendations !== 'CONNF404' && targetArea) {
// Display the personalized recommendations
} else {
// Display the default recommendations
displayDefaultContent(products, targetArea);
}
}
- When you configure the personalized zones using custom attributes, add the
headerElement
attribute:
personalizationObject.onReady(function(){
var zones = document.querySelectorAll('[custom-pzn]');
zones.forEach(function(value) {
var zoneObj = {
"zoneId": value.getAttribute('custom-pzn-zone-reg-id'),
"targetArea": value.getAttribute('custom-pzn-target-area'),
"num": value.getAttribute('custom-pzn-product-number'),
“headerElement”: value.getAttribute('custom-pzn-header-id')
};
var validElement = validateDOMElement(zoneObj.targetArea)
if(validElement) {
personalizationObject.getContent(zoneObj)
.then(function(result) {
personalizationHandler(result, validElement, zoneObj.headerElement)
});
}
});
});
For HTML code snippet, make the following changes.
<div>
<h3 id="HEADER_ELEMENT_ID"> Custom header text will be displayed here </h3>
<div id="<ZONE_ID>" wrtp-recommended-zone="<ZONE_ID>"
custom-pzn
custom-pzn-zone-reg-id="<ZONE_ID>"
custom-pzn-target-area="<ZONE_ID>[CAN_BE_SAME_AS_<ZONE_ID>]"
custom-pzn-product-number="<NUMBER_OF_PRODUCTS_REQUIRED>[OPTIONAL]>"
custom-pzn-header-id="<HEADER_ELEMENT_ID>"
</div>
<!--Replace HEADER ELEMENT ID with ID of DOM element used to display the html header-->
</div>
If you configure personalized zones without using custom attributes, make the following code changes.
personalizationObject.onReady(function(){
var zones = [
{
"zoneId": "ZONE_ID_1",
"targetArea": "ELEMENT_ID_2",
"num": "NUMBER_OF_PRODUCTS_[OPTIONAL]",
“headerElement”: "HEADER_ELEMENT_ID_1 [OPTIONAL]"
},
{
"zoneId": "ZONE_ID_2",
"targetArea": "ELEMENT_ID_2",
"num": "NUMBER_OF_PRODUCTS_[OPTIONAL]",
“headerElement”: "HEADER_ELEMENT_ID_2 [OPTIONAL]"
},
]
zones.forEach(function(zoneObj) {
var validElement = validateDOMElement(zoneObj.targetArea)
if(validElement) {
personalizationObject.getContent(zoneObj)
.then(function(result){
personalizationHandler(result, validElement, zoneObj.headerElement)
});
}
});
});
For HTML code snippet, make the following change:
<div>
<h3 id="HEADER_ELEMENT_ID"> Custom header text will be displayed here </h3>
<!-- Replace HEADER_ELEMENT_ID with the header ID-->
<div id="ZONE_ID" wrtp-recommended-zone="ZONE_ID">
<!-- Replace ZONE_ID with the registered zone ID-->
</div>
</div>
The following code sample summarizes the changes to display the recommendation header.
// Step 1
<script type="text/javascript">
. . .
// Helper functions for personalizationHandler
. . .
. . .
var displayRecommendations = function (products, targetArea, headerElementId) {
var recommendations = products.value;
if (products.header && headerElementId) {
document.getElementById(headerElementId).innerHTML = products.header;
}
if (recommendations && recommendations !== 'CONNF404' && targetArea) {
// Display the personalized recommendations
} else {
// Display the default recommendations
displayDefaultContent(products, targetArea);
}
}
// Step 6
// A handler at the client side to process the results
var personalizationHandler = function(result, targetArea, headerElement)
{
switch(result.type) {
case "recommendation":
displayRecommendations(result, targetArea, headerElement);
break;
case "custom-url":
displayCustomUrl(result, targetArea);
break;
. . .
. . .
}
}
// Step 7
// Configure personalization
personalizationObject.onReady(function(){
// Step 8 configure zones (Approach 1)
var zones = document.querySelectorAll('[custom-pzn]');
zones.forEach(function(value) {
var zoneObj = {
"zoneId": value.getAttribute('custom-pzn-zone-reg-id'),
"targetArea": value.getAttribute('custom-pzn-target-area'),
"num": value.getAttribute('custom-pzn-product-number'),
“headerElement”: value.getAttribute('custom-pzn-header-id')
};
var validElement = validateDOMElement(zoneObj.targetArea)
if(validElement) {
personalizationObject.getContent(zoneObj)
.then(function(result) {
personalizationHandler(result, validElement, zoneObj.headerElement)
});
//Optional code to capture click events
personalizationObject.collectBehavior("ELEMENTID","EVENT ","ZONEID");
}
});
});
} else {
console.log("Error in loading the Personalization library.");
}
});
</script>
Recommendation title for Angular SPA channel zone
Install library version 2.2.0 on your Angular SPA channel.
Zone configuration
- Configure Angular SPA zone for product recommendations. For more information, see
- In the "personalizationHandler" function, add the following code changes:
function personalizationHandler(result, headerElement) {
switch(result.type) {
case "recommendation":
return displayRecommendations(result, headerElement);
case "custom-url":
return displayCustomUrl(result);
case "custom-html":
return displayCustomHtml(result);
case "wch-url":
return displayWchUrl(result);
case "wch-html":
return displayWchHtml(result);
case "":
return displayDefaultContent(result);
}
}
- Also, modify the
displayRecommendations()
function in thepersonalizationHandler()
function as shown:
function displayRecommendations(products, headerElement) {
let recommendations = products.value;
if (products.header && headerElement) {
document.getElementById(headerElement).innerHTML = products.header;
}
if (recommendations && recommendations !== "CONNF404") {
// return the personalized recommendations
} else {
// return the default recommendations
return displayDefaultContent(products);
}
}
- Modify the
VARIABLE_NAME = personalizationHandler(result);
as follows:
this.personalizationObject.onReady(()=>{
this.personalizationObject.getContent({ zoneId:‘<ZoneRegId>’, num: <numberofproducts>[OPTIONAL]})
.then(
result => {
this.VARIABLE_NAME = personalizationHandler(result, ‘<HeaderElementId>’[OPTIONAL]);
// Use VARIBLE_NAME to display the contents
}
)
});
- Modify the HTML snippet as shown here.
<h3 id="<HeaderElementId>"> Custom header text will be displayed here </h3>
//Replace HeaderElementID with the ID of DOM element used to display custom header
<div id="<ZoneRegId>" wrtp-recommended-zone="<ZoneRegId>">
<div *ngIf="<VARIABLE_NAME>"></div> // Use this variable to display and render the contents
</div>
Recommendation title for React SPA channel zone
Install library version 2.2.0 on your React SPA channel.
Zone configuration
- Configure React SPA zone for product recommendations. For more information, see
- In the
"personalizationHandler"
function, add the following code changes:
componentDidMount() {
this.personalizationObject = this.context;
function personalizationHandler(result, headerElement) {
switch(result.type) {
case "recommendation":
return displayRecommendations(result, headerElement);
case "custom-url":
return displayCustomUrl(result);
case "custom-html":
return displayCustomHtml(result);
case "wch-url":
return displayWchUrl(result);
case "wch-html":
return displayWchHtml(result);
case "":
return displayDefaultContent(result);
}
}
In the step definition of the helper functions, make the following changes.
function displayRecommendations(products, headerElement) {
let recommendations = products.value;
if (products.header && headerElement) {
document.getElementById(headerElement).innerHTML = products.header;
}
if (recommendations && recommendations !== "CONNF404") {
// return the personalized recommendations
} else {
// return the default recommendations
return displayDefaultContent(products);
}
}
- Modify the
<VARIABLE_NAME>: personalizationHandler(result);
as follows:
this.personalizationObject.onReady(() => {
this.personalizationObject.getContent({zoneId : '<ZoneRegId>', num: <numberofproducts>[OPTIONAL]})
.then(result =>{ this.setState({
<VARIABLE_NAME>: personalizationHandler(result, ‘<headerElement_Id>’)
// Replace <VARIABLE_NAME> with personalized content.
// Replace <headerElement_Id> with the Id of the DOM element that is responsible for displaying custom header text for the zone.
});
});
});
Your marketer can now specify the title for the recommendation zone when they create a product recommendation strategy.
Updated over 2 years ago