Capture iframes from other domains (cross-domain)
The Connect web library can capture HTML pages nested into iframes.
<iframe src="form.html" width="400" height="200"></iframe>
Preconditions
For iframe capturing, the captureFrames
property must be set to true
. This is the default value in the library configuration.
domCapture: {
diffEnabled: true,
options: {
maxLength: 10000000,
captureShadowDOM: true,
captureDynamicStyles: true,
captureFrames: true,
debugVoidElementsEnabled: false,
debugVoidElementsTimer: 10000
}
}
If the iframe is served from the same domain (including the subdomain), no further configuration is required. If the iframe is served cross-domain, from a different subdomain or another domain, some additional configuration is necessary.
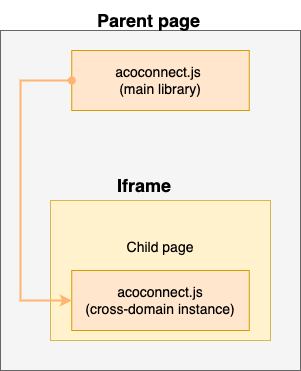
How it works
When the iframe is served from the same domain, the library can capture its contents directly, and only needs to be deployed on the parent page.
When the iframe is served cross-domain, from a different subdomain or another domain, a copy of the library must be deployed in both the parent and the child page served through the iframe. If more than one cross-domain iframe needs to be captured, the library must be deployed in all child pages. The library will be configured slightly differently in the parent and in the child page.
Follow the instructions below to enable cross-domain iframe capture.
Instructions for configuring the SDK in the parent and child pages
Step A: Update the parent page
Configure the Connect library as follows on the parent page (the one containing the iframe).
let config = window.TLT.getDefaultConfig()
// Enable for cross-domain communication
config.core.frames.enableCrossDomainCommunication = true
// Optional. If you want to whitelist a certain domain, use following property. Otherwise the library will capture all domains automatically.
config.core.frames.eventConsumer.childFrameHostnameWhitelist.push("<<website.com>>"")
// Initialize the library with updated configuration.
window.TLT.initLibAdv("<<appKey>>", "<<collectorPostURL>>", config, true, true, true, true, true, undefined)
Here is the minimal HTML source that includes the JavaScript above.
<html>
<head>
<!--Use the latest library-->
<script src="https://cdn.goacoustic.com/connect/latest/acoconnect.js"></script>
<script type="text/javascript">
let config = window.TLT.getDefaultConfig()
config.core.frames.enableCrossDomainCommunication = true
// If you want to whitelist a certain domain, add the following property:
// config.core.frames.eventConsumer.childFrameHostnameWhitelist.push("website.com")
// Initialize the library with updated configuration.
window.TLT.initLibAdv("< < Your App Key >> ", " < < You Collector URL >> ", config, true, true, true, true, true, undefined)
</script>
</head>
<body>
<h1>Main page</h1>
<iframe src="http://mywebsite.com/index1.html" width="600" height="200"></iframe>
<iframe id="frame2" src="http://mywebsite.com/index2.html" width="600" height="200"></iframe>
<iframe id="my-iframe" src="http://anotherWebsite.com/index.html" width="600" height="400"></iframe>
</body>
</html>
Step B: Update the child page
Configure the Connect library as follows on the child page being served through the iframe.
let config = window.TLT.getDefaultConfig()
// Enable for cross-domain communication
config.core.frames.enableCrossDomainCommunication = true
// In order to adjust path and IDs for data, indicate the ID of the iframe to be used on parent page.
config.core.frames.eventProducer.producerId = "<<myIframeId>>"
// Initialize the library with updated configuration
window.TLT.initLibAdv("<<Your App Key>>", "<<You Collector URL>>", config, true, true, true, true, true, undefined)
Here is the minimal HTML source that includes the JavaScript above.
<html>
<head>
<!--Use the latest library-->
<script src="https://cdn.goacoustic.com/connect/latest/acoconnect.js"></script>
<script type="text/javascript">
let config = window.TLT.getDefaultConfig()
config.core.frames.enableCrossDomainCommunication = true
config.core.frames.eventProducer.producerId = "my-iframe"
window.TLT.initLibAdv("<<Your App Key>>", "<<You Collector URL>>", config, true, true, true, true, true, undefined)
</script>
</head>
<body>
Iframe page
</body>
</html>
Browser limitations
The Connect web library uses the postMessage
API to communicate with the cross-domain iframe. This solution does not work for browsers that do not support the postMessage
API.
For more information, see Cross-document messaging and Same-origin policy.
Updated 3 days ago