Integrate the Tealeaf SDK into your iOS app
You can integrate the Tealeaf SDK into native iOS applications build on Swift, SwiftUI and Objective-C.
Note
New to Tealeaf? Install our preconfigured sample app and learn the implementation faster.
Requirements
- Acoustic Tealeaf. To use the library, your company must have an active Tealeaf subscription.
- Development environment. You can integrate the Tealeaf SDK into native iOS applications build on Swift, SwiftUI and Objective-C. You will need Xcode 15 with Command Line Tools.
- Mobile app compatibility. The library can capture user experience data on end users' devices running iOS 13 and later. On iPadOS, only single-window applications are currently supported.
Warning
We offer limited support for iPad apps with a multi-window architecture. Tealeaf captures data from such apps and makes it available for analytics, but session replays may not work correctly.
Prerequisites
If your project is built on SwiftUI, make sure it contains AppDelegate.
Beta vs. release version
If you haven't used the Tealeaf SDK before, we highly recommend starting with the beta version (TealeafDebug). It contains debug information.
Here is how to enable debug logs in your Xcode console window:
- Use the beta version during the initial setup.
- Update your project scheme by adding two environment variables with the value of
1
:EODebug
andTLF_DEBUG
.
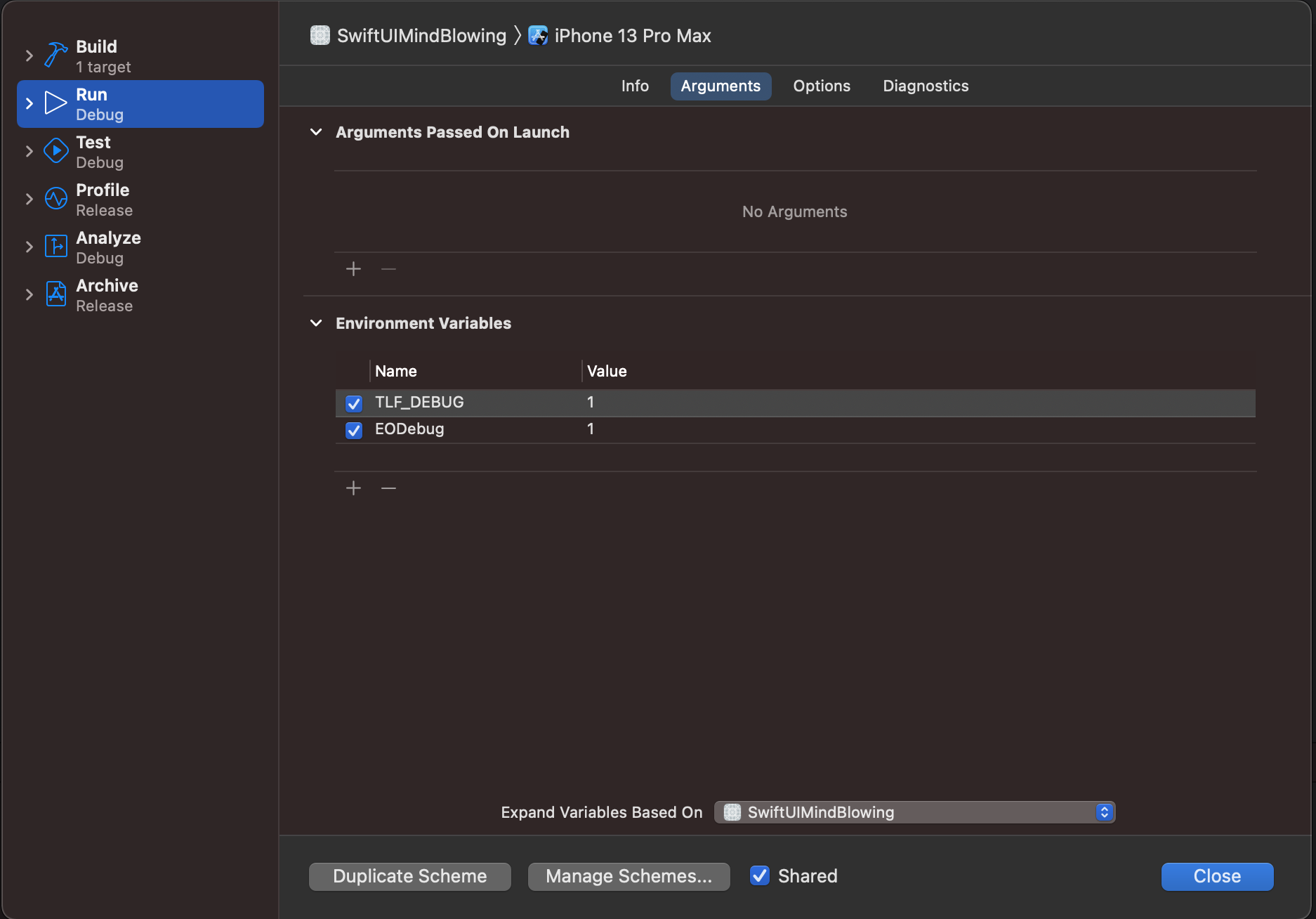
Important:
- Never use the release and beta builds together in the same project.
- When reporting an issue to our support team, always attach your debug log. It speeds up troubleshooting.
Initial setup
The easiest way to install the Tealeaf SDK is using a dependency manager. A manual option is also available - see Manually integrate the Tealeaf SDK into your iOS app.
You can add the Tealeaf SDK to your iOS app using the CocoaPods dependency manager. This option requires a recent version of CocoaPods.
Here are steps to follow:
- If you don't have a Podfile in your Xcode project directory, create one.
- Open the Podfile in a text editor and make sure the iOS version is 12.0 or later. Then uncomment
use_frameworks!
. - In the same Podfile, set
pod
to eitherTealeaf
orTealeafDebug
, depending on the build you want to use.
source 'https://github.com/CocoaPods/Specs.git'
platform :ios, '12.0'
target 'SwiftUIMindBlowing' do
use_frameworks!
pod 'TealeafDebug'
end
- In a terminal emulator, navigate to your project directory.
- Install the pods. Make sure the command is completed with no errors. If you get an error, run the same command with the
--verbose
option and share the error log with our services team.
pod install
Important notes:
- Sometimes CocoaPods fails to load the latest version. So you may need to pull it using
pod update
. - Use the
$(inherited)
flag in your application target's "Other Linker Settings". This will ensure all the Pods are linked correctly.
You can add the Tealeaf iOS SDK to your app using the Carthage dependency manager.
- Create a Cartfile in your Xcode project directory if you don't have one yet.
touch Cartfile
- Open the Cartfile in a text editor and add the following lines for the release build. To use the beta build, update the file names: instead of Tealeaf.json use TealeafDebug.json and instead of EOCore.json use EOCoreDebug.json.
binary "https://raw.githubusercontent.com/acoustic-analytics/Tealeaf/master/Tealeaf.json"
binary "https://raw.githubusercontent.com/acoustic-analytics/EOCore/master/EOCore.json"
- From the root project directory, run the command
carthage update --use-xcframeworks
. Make sure it completes without errors. - In a file manager, navigate to
/Carthage/Build
inside your project directory and select two bundles:EOCore.xcframework
andTealeaf.xcframework
.
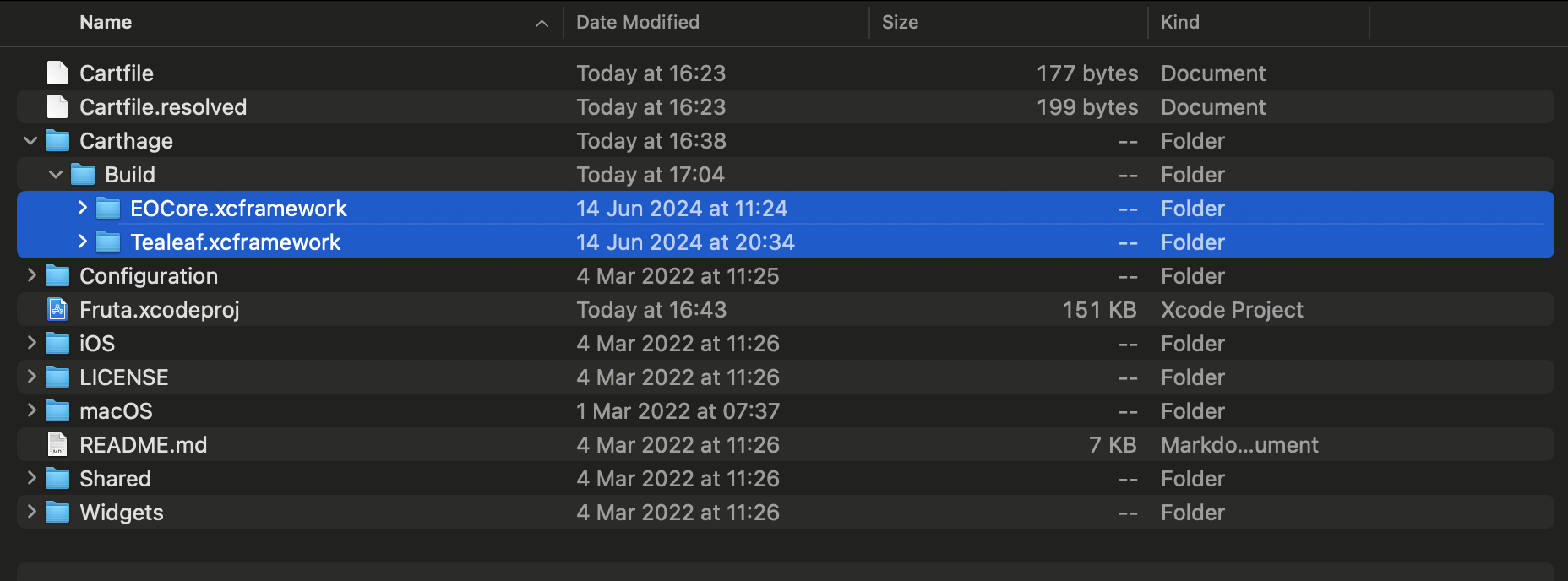
- Select the primary project target. Drag and drop the bundles to Frameworks, Libraries, and Embedded Content in the target settings. Select Embed & Sign for each bundle.
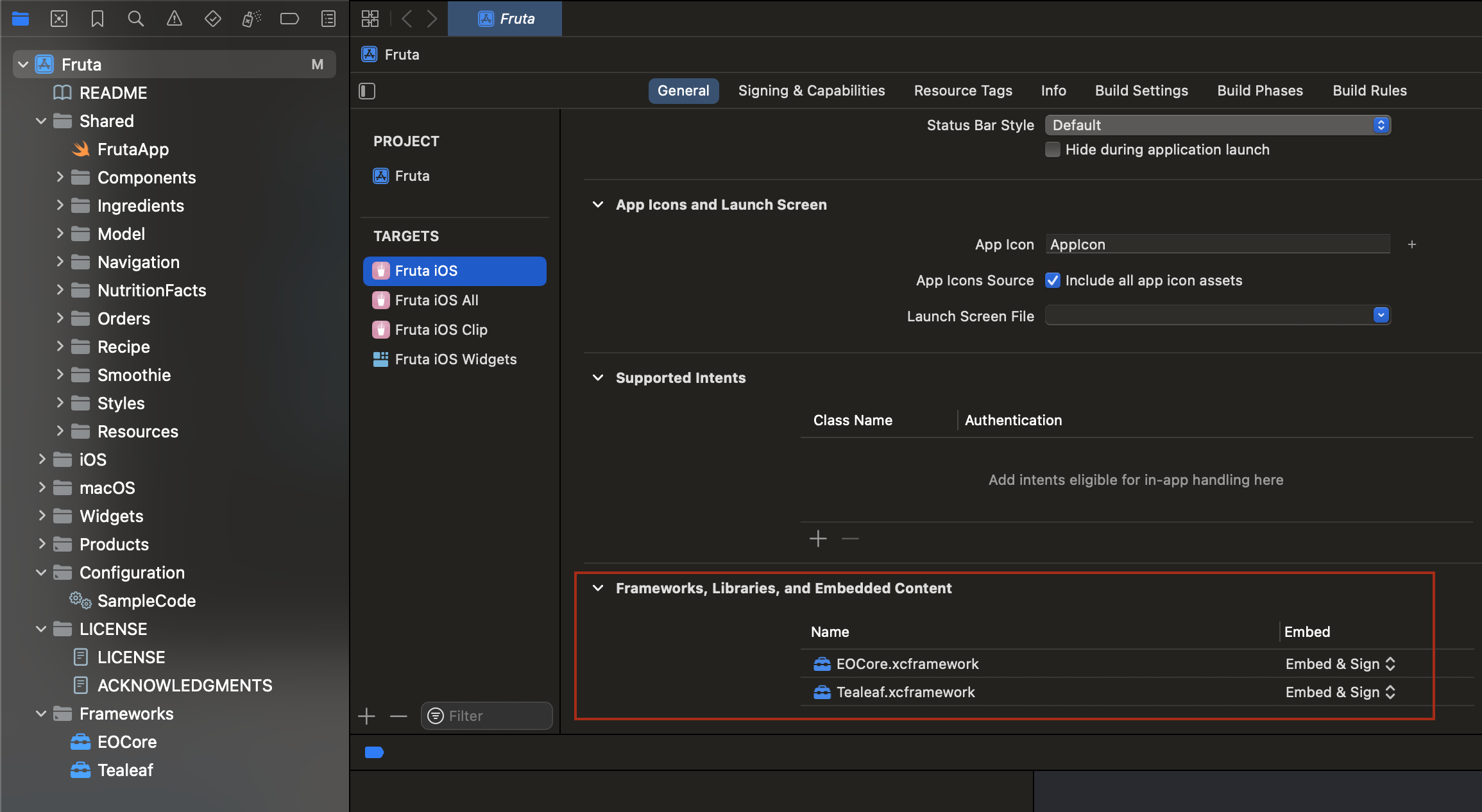
- Switch to the Build Phases tab and make sure the bundles appear under Embed Frameworks. If they don't, you will need to add them as frameworks.
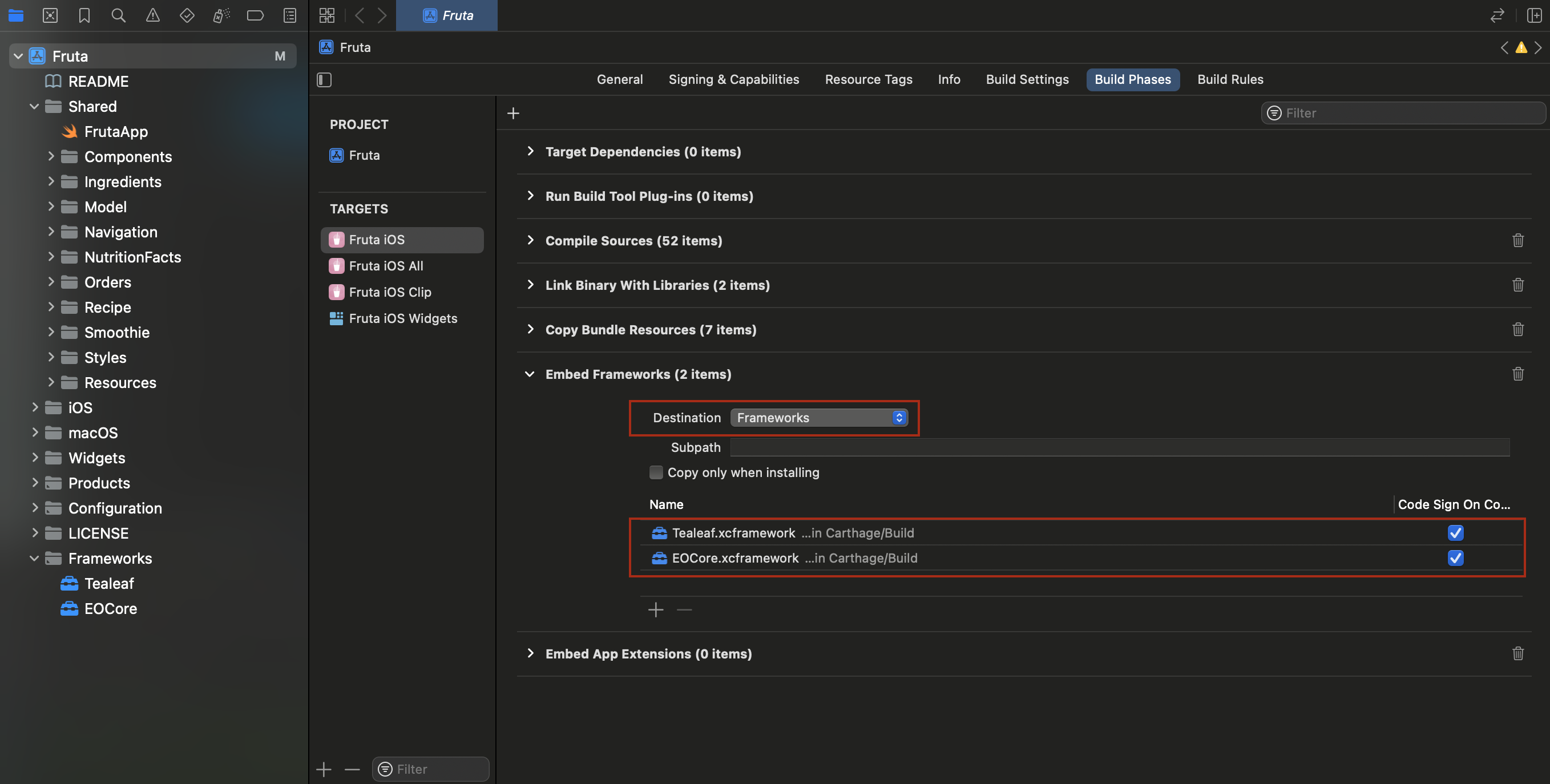
- Rebuild the project.
You can add the Tealeaf iOS SDK as a Swift Package.
- In your Xcode project, go to File > Add Package Dependencies.
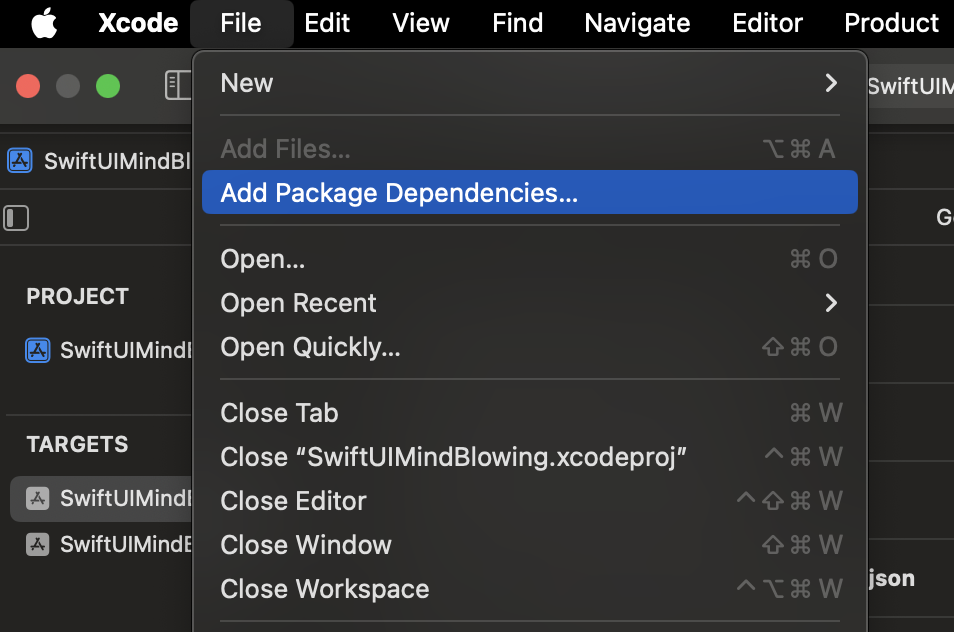
- Search for
https://github.com/go-acoustic/Tealeaf-SP
. For the beta build, replaceTealeaf-SP
withTealeafDebug-SP
.
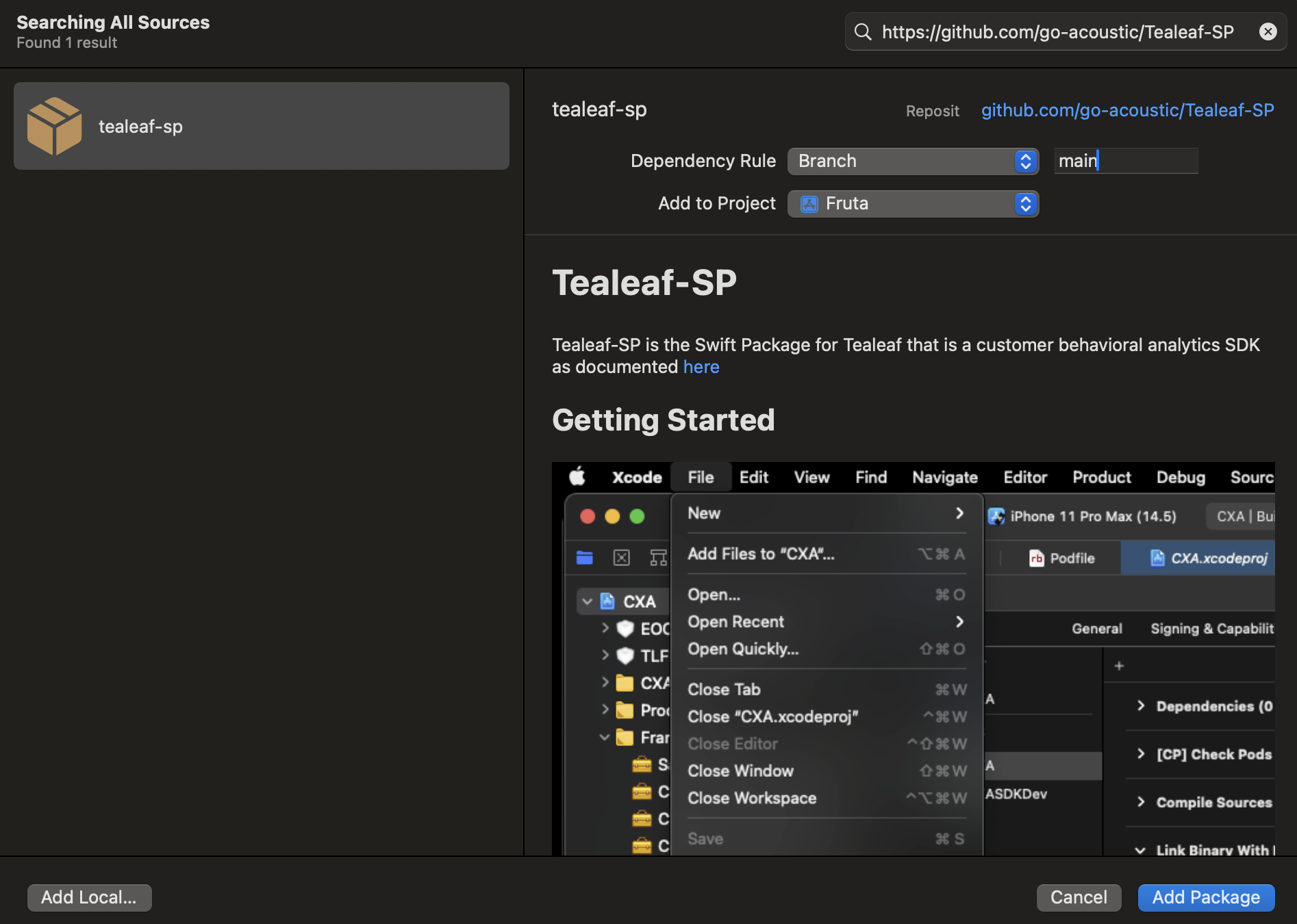
- Select the target to add the library to.
Required configuration
- Open the AppDelegate.swift file and add 2 import statements there.
import EOCore
import Tealeaf
- Add the Tealeaf framework to the AppDelegate class. In the example below,
app_key
is the application key andendoint_url
is the endpoint URL assigned to your organization. You must replace these with your own credentials.
TLFApplicationHelper().enableTealeafFramework(
"app_key",
withPostMessageUrl: "endpoint_url")
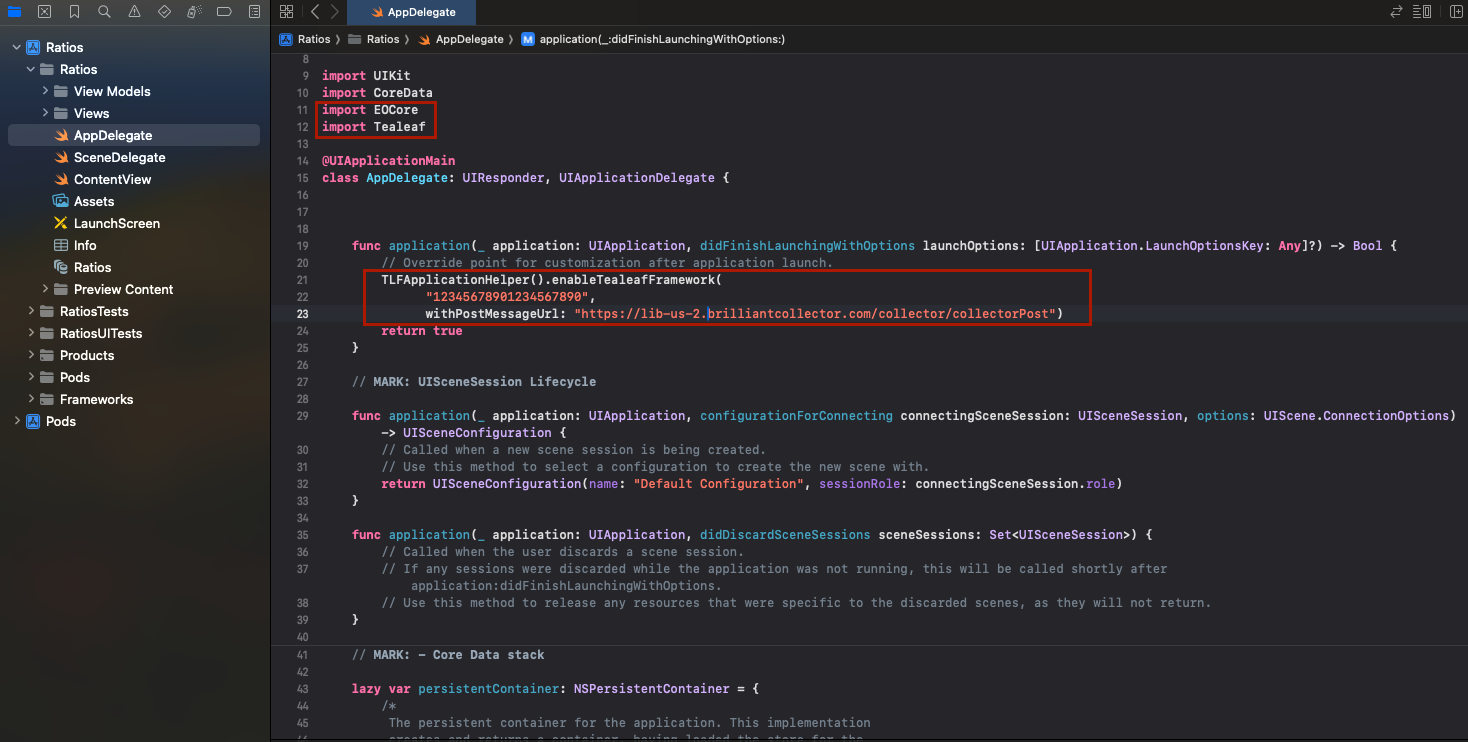
- (optional) If you want your application to capture the identifier for advertisers (IDFA) data using the Tealeaf
AdvertisingId
property, add the following snippet to the AppDelegate class:
TLFApplicationHelper.sharedInstance().setCXAAdvertisingId(
ASIdentifierManager.shared().advertisingIdentifier.uuidString)
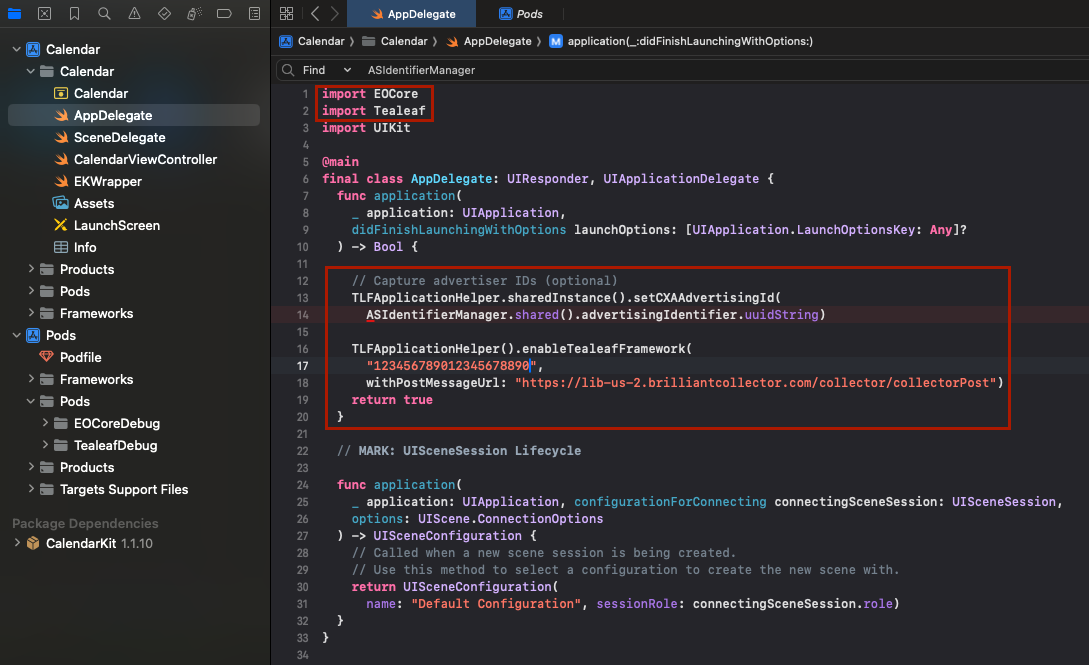
- Build and run the application.
- Open the AppDelegate.m file in your project.
- Add the Tealeaf import statement to the header.
@import Tealeaf;
- Add the following code to the
didFinishLaunchingWithOptions
function. Replaceapp_key
andendpoint_url
with your Tealeaf credentials.
[[TLFApplicationHelper sharedInstance] enableTealeafFramework:@"app_key" withPostMessageUrl:@"endpoint_url"];
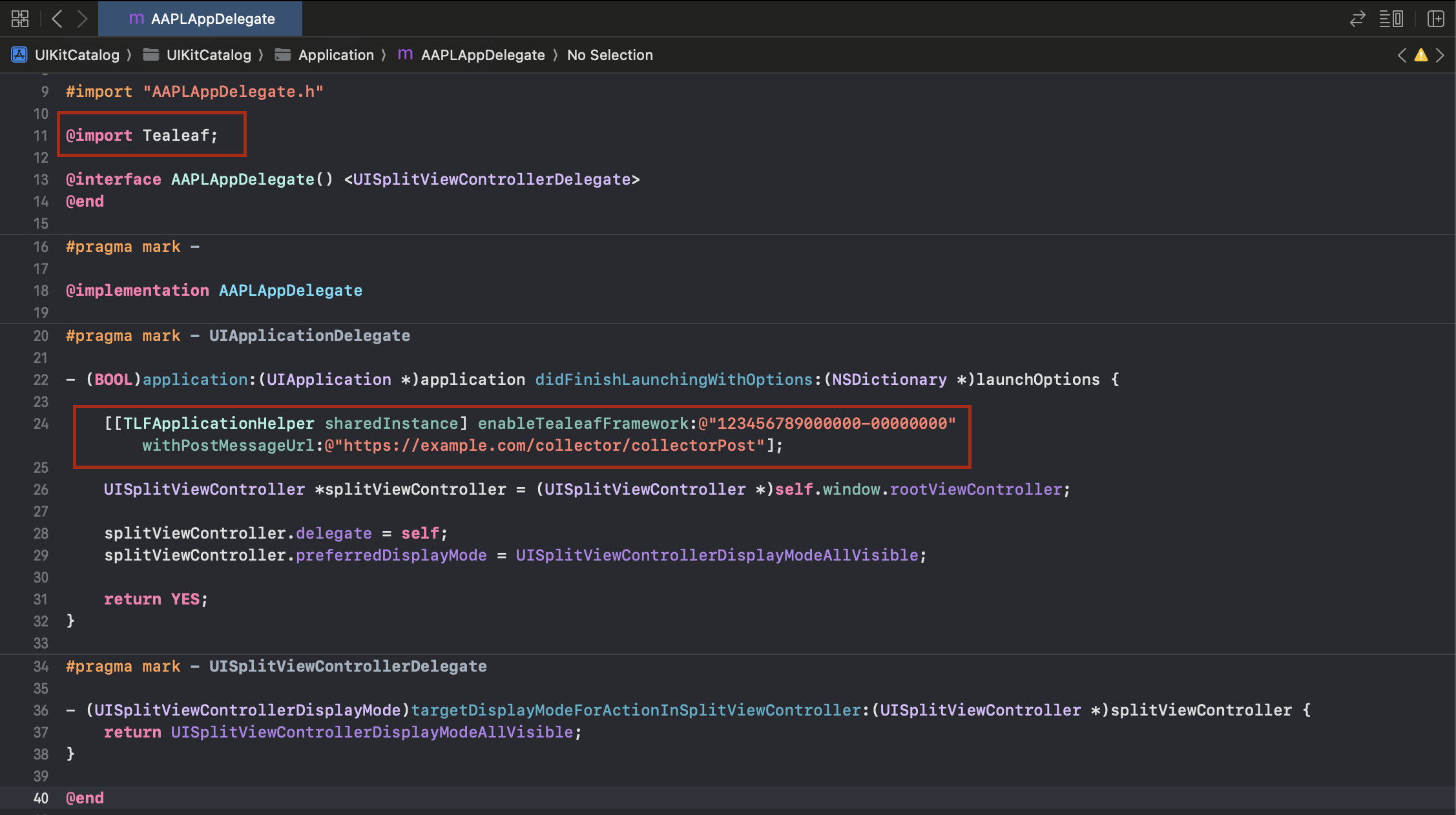
- (optional) If you want your application to capture Apple identifier for advertisers (IDFA) data, add an additional snippet to AppDelegate.m.
TLFApplicationHelper.sharedInstance().setCXAAdvertisingId(
ASIdentifierManager.shared().advertisingIdentifier.uuidString)
- Build and run the application.
Further steps
- To verify the integration, click around in your app and then find the session in Tealeaf. For details, see Session replay in our support center.
- Make sure the library doesn't capture visitors' personally identifiable information (PII). To learn more, see Enable privacy protection in the Tealeaf iOS SDK.
Updated 4 months ago