Integrate the Campaign Cordova SDK into your app
Cordova Campaign SDK provides mobile app message handling and display capabilities for Android and iOS apps. To add the SDK to your app, use npm CLI. For basic and advanced settings, we provide a unified configuration file CampaignConfig.json.
Warning
The Campaign SDK does not encrypt data transmitted via push notifications and in-app messages. To transmit sensitive information, you must encrypt it before sending and decrypt it in the memory of the end user's device.
Requirements
Acoustic Campaign
- To use the SDK, your company must have an active Acoustic Campaign subscription.
- You must have a developer account for Acoustic Campaign. The account must have the following permissions enabled: Enable Mobile App Push and Enable Development for Mobile App Push.
- You must have the base URL associated with your subscription. Contact your account manager if you don't have the base URL yet.
Mobile app compatibility
The Campaign library works on end users' devices running the following operating systems:
- Android 5.0 (API level 21) and later
- iOS 13 and later
Warning
The iOS Simulator app is unable to handle push messages. Run your app on a real device.
Development environment
To integrate the Campaign SDK into an iOS app using Cordova, you will need the following:
- Xcode 15 with Command Line Tools
- Cordova iOS plugin 7.1.x or later
- Apple Developer Program membership (free accounts do not support push notifications)
To integrate the Campaign SDK into an Android app using Cordova, you will need the following:
- Android Studio Iguana | 2023.2.1 Patch 1 or later
- Cordova Android plugin 12.0.1 or later
See official Cordova documentation for detailed requirements.
Before you begin
Before you begin, make sure the following conditions are met.
- Your iOS project uses an explicit App ID and is assigned to a team. For the App ID, the push notification service is enabled.
- In your iOS project, the Push Notifications capability is enabled. For instructions, see Adding capabilities to your app in the Xcode guide.
- Your app is registered with Apple Push Notification service (APNs). You can use either of the following authentication methods to secure connection to APNs: token-based (p8) (recommended by Apple) or certificate-based (p12).
Important
The provisioning profile must match the push certificate. For example, a development provisioning profile works with a development push certificate.
What to prepare for the setup depending on the selected authentication type:
Authentication type | Credentials to submit to Acoustic Campaign |
---|---|
Token-based (p8) | 1) An authentication token signing key, which is a text file with the .p8 extension 2) A 10-character string with the Key ID 3) The Team ID associated with your app 4) The Bundle ID assigned to your app |
Certificated-based (p12) | 1) A certificate file with the .p12 extension 2) CSR (a private key used to encrypt the certificate) |
- Your mobile app is registered and implemented (this is necessary to enable testing and creating notifications).
- Firebase Cloud Messaging is enabled.
- The install location of your app must be set to the internal memory of a mobile device, not to external storage cards.
Initial setup
Navigate to the main project directory and add cordova-acoustic-mobile-push
.
$ npm i cordova-acoustic-mobile-push
If you need to use a beta or debug build, add -beta to the name of the package.
$ npm i cordova-acoustic-mobile-push-beta
Then run the following Node.js command from the main project directory. It will apply the changes and install related frameworks.
node node_modules/cordova-acoustic-mobile-push-sdk/scripts/installPlugins.js
Customizing library configuration
Step A: get an app key
To activate the Campaign SDK within your iOS app, you must generate an app key for it and upload the app's push certificate to Acoustic Campaign.
- Log in to Acoustic Campaign under a developer account.
- In the navigation menu, select Administration > Push developer resources.
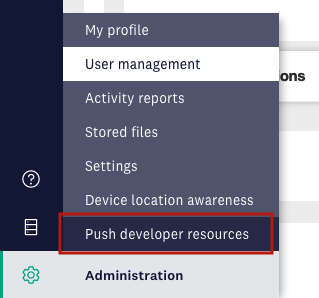
- Under Apps, click to add a new app.
- In the Push Service field, select APNs. Then select the type of authentication to use: P8 or P12. Provide required credentials for the selected authentication type.
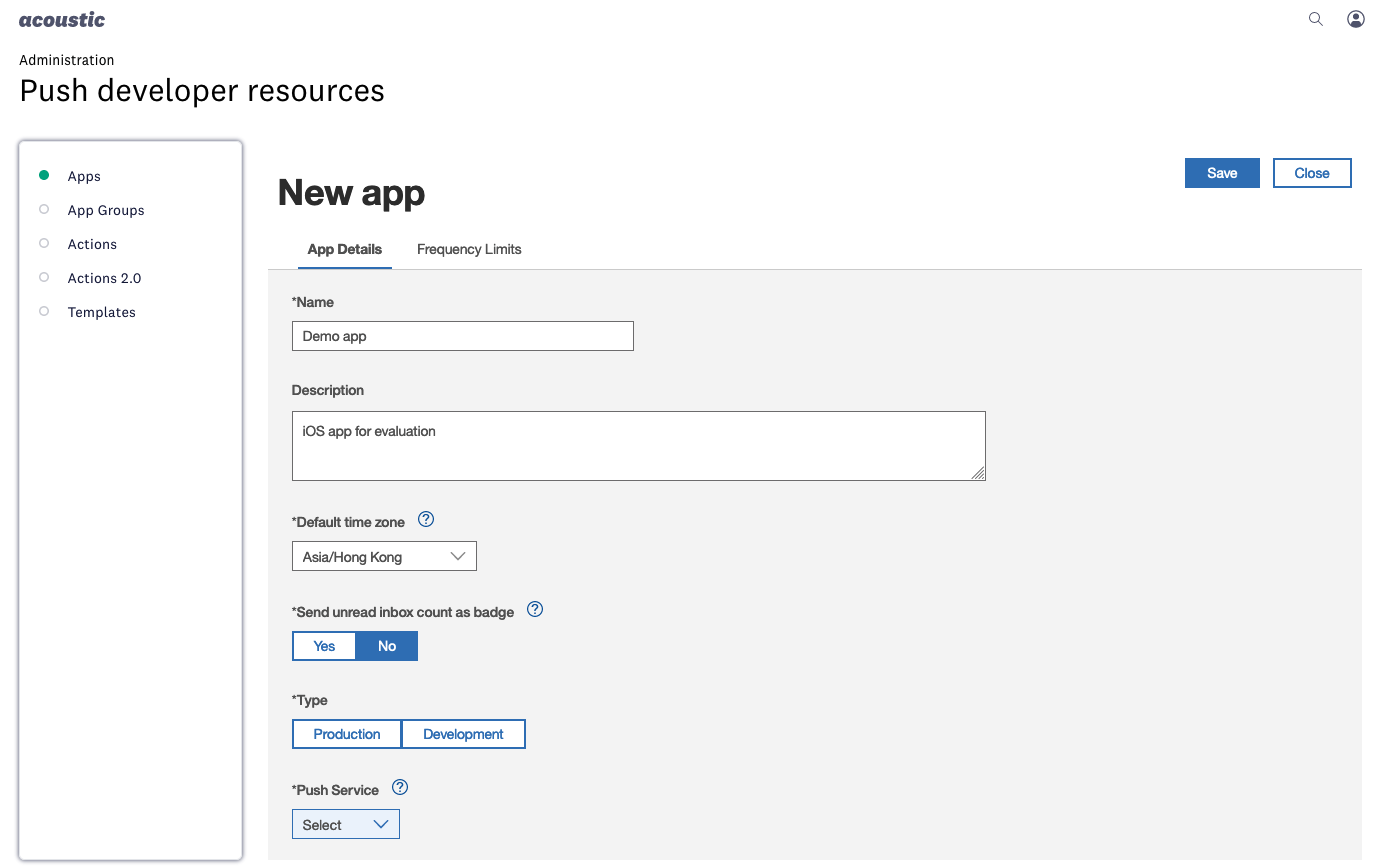
- Set the Type setting in accordance with the distribution model. Select Development to run the app from Xcode with a development provisioning profile. Or select Production to run the app with an App Store, Enterprise, or ad hoc provisioning profile.
- Fill out the remaining settings and save the changes.
- Copy your app key and keep it handy for the setup.
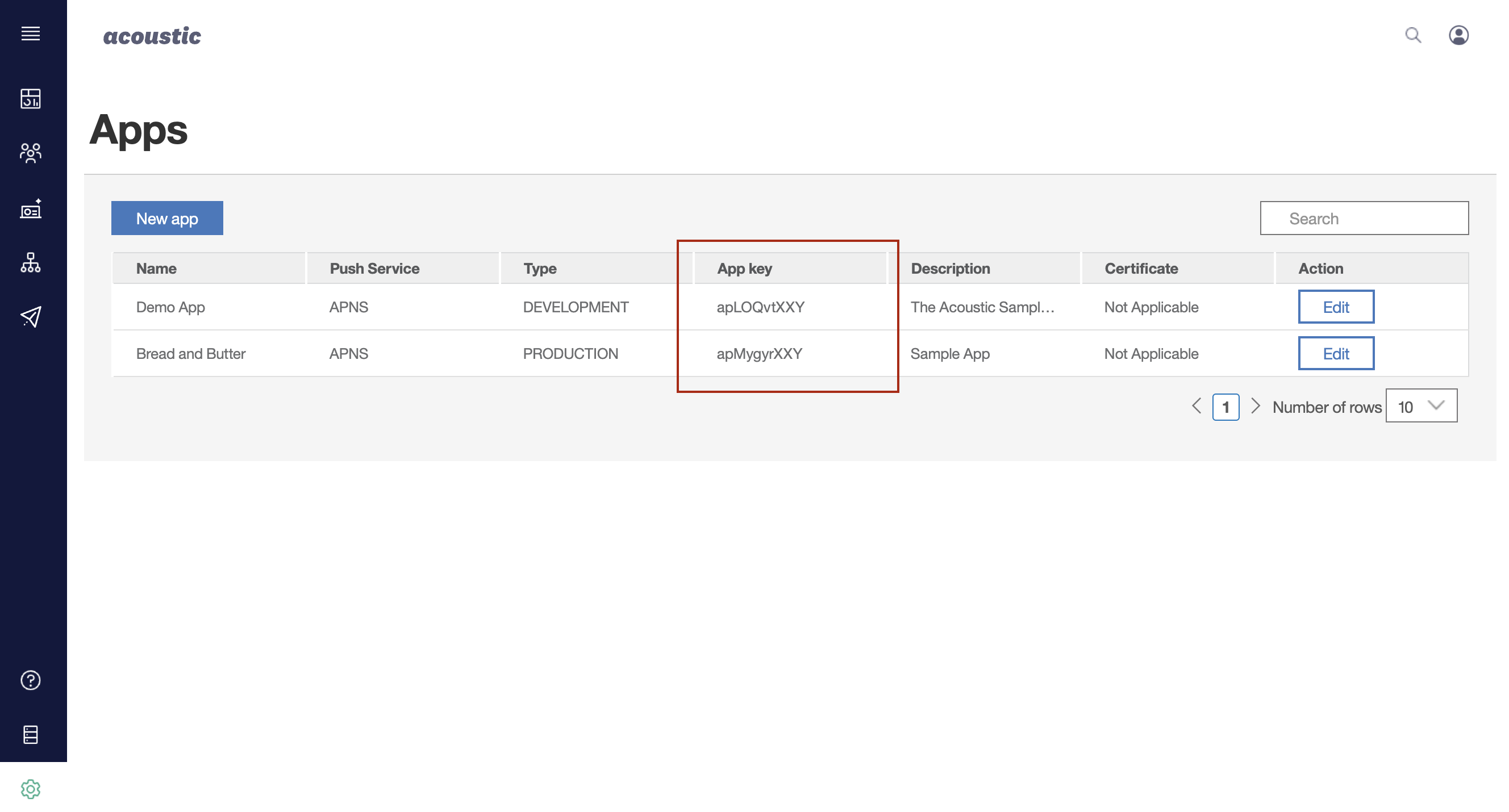
Step B: update the configuration file
Find a new file (CampaignConfig.json) in the root directory of your app. Use this file to customize the library configuration.
- Make sure you are using the right build. During testing, set
useRelease
tofalse
. For production, usetrue
.
{
"useRelease": true
}
- Check the version of the Campaign SDK for your iOS app. You can enter a version number or submit an empty value to use the latest available version (recommended).
{
"iOSVersion": ""
}
- In the
iOS
section, enter theappKey
you have generated and thebaseUrl
provided by your account manager. Do not change the app key after you publish your app.
{
"iOS": {
"baseUrl": "https://mobile-sdk-lib-XX-Y.brilliantcollector.com",
"appKey": {
"dev": "INSERT YOUR DEV APPKEY HERE",
"prod": "INSERT YOUR PROD APPKEY HERE"
}
}
}
- Important: to apply the changes, run the following Node.js command from the main project directory.
node node_modules/cordova-acoustic-mobile-push-sdk-beta/scripts/installPlugins.js
To learn more about your options, see the following recipe: Configure mobile SDKs [iOS].
Step A: get an app key
To activate the Campaign SDK within your iOS app, you must generate an app key for it and upload the app's push certificate to Acoustic Campaign.
- Log in to Acoustic Campaign under a developer account.
- In the navigation menu, select Administration > Push developer resources.
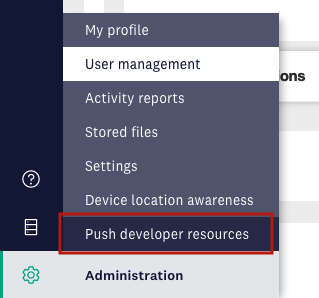
- Under Apps, click to add a new app.
- In the Push Service field, select GCM/FCM. Set the type of authentication to V1. Provide required credentials for the selected authentication type.
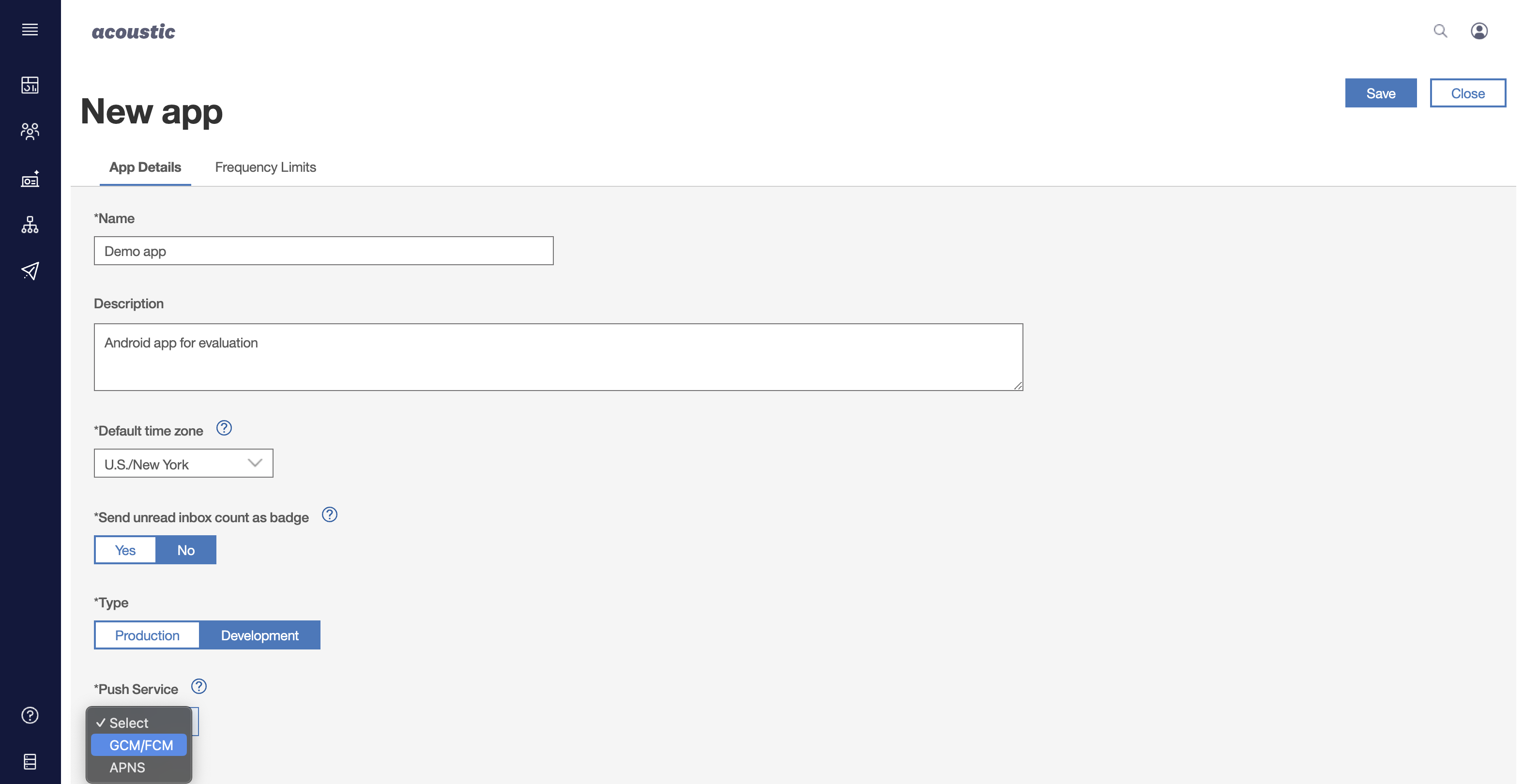
- Fill out the remaining settings and save the changes.
- Copy your app key and keep it handy for the setup.
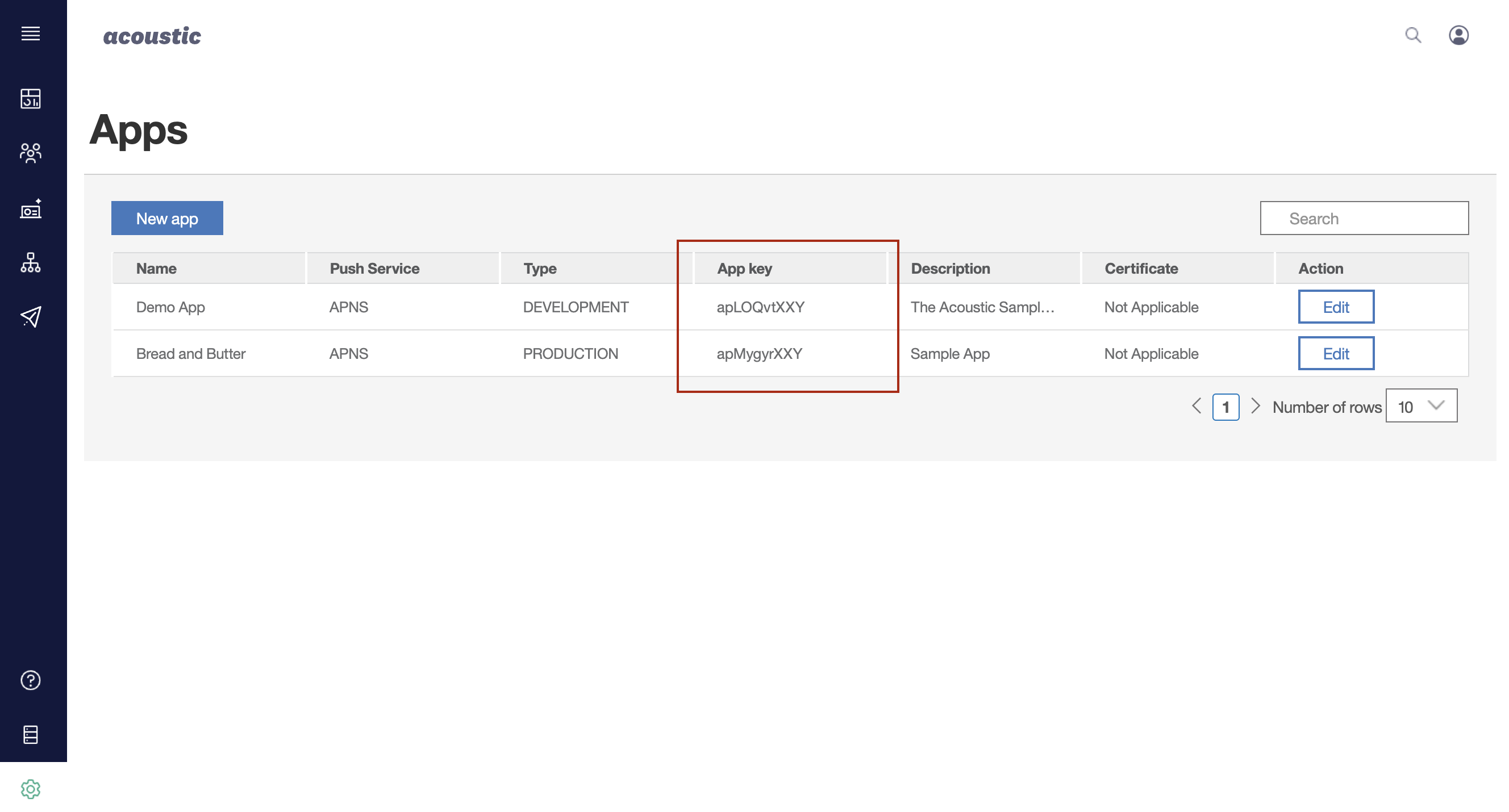
Step B: update the configuration file
Find a new file (CampaignConfig.json) in the root directory of your app. Use this file to customize the library configuration.
- Make sure you are using the right build. During testing, set
useRelease
tofalse
. For production, usetrue
.
{
"useRelease": true
}
- Check the version of the Campaign SDK for your Android app. You can enter a version number or submit an empty value to use the latest available version (recommended).
{
"androidVersion ": ""
}
- In the
android
section, enter theappKey
andbaseUrl
provided by your account team. Do not change the app key after you publish your app.
{
"android": {
"baseUrl": "https://mobile-sdk-lib-XX-Y.brilliantcollector.com",
"appKey": {
"prod": "INSERT APP KEY HERE"
}
}
}
- Important: to apply the updates, run the following Node.js command from the main project directory.
node node_modules/cordova-acoustic-mobile-push-sdk-beta/scripts/installPlugins.js
To learn more about your options, see the following recipe: Configure mobile SDKs [Android].
Final project preparation
Step A: Add the Notification Service Extension
One of the frameworks installed using the installPlugins.js script is AcousticMobilePushNotification. It lets you enable dynamic action categories and media attachments in iOS notifications. Before a notification from the APNs service is displayed to an end user, the media attachment URL is extracted, and then the media file is downloaded and attached to the notification.
The notification service extension functions as a separate app within your app bundle and requires separate provisioning.
- In your XCode project, click File > New > Target.
- On the iOS tab, select Notification Service Extension.
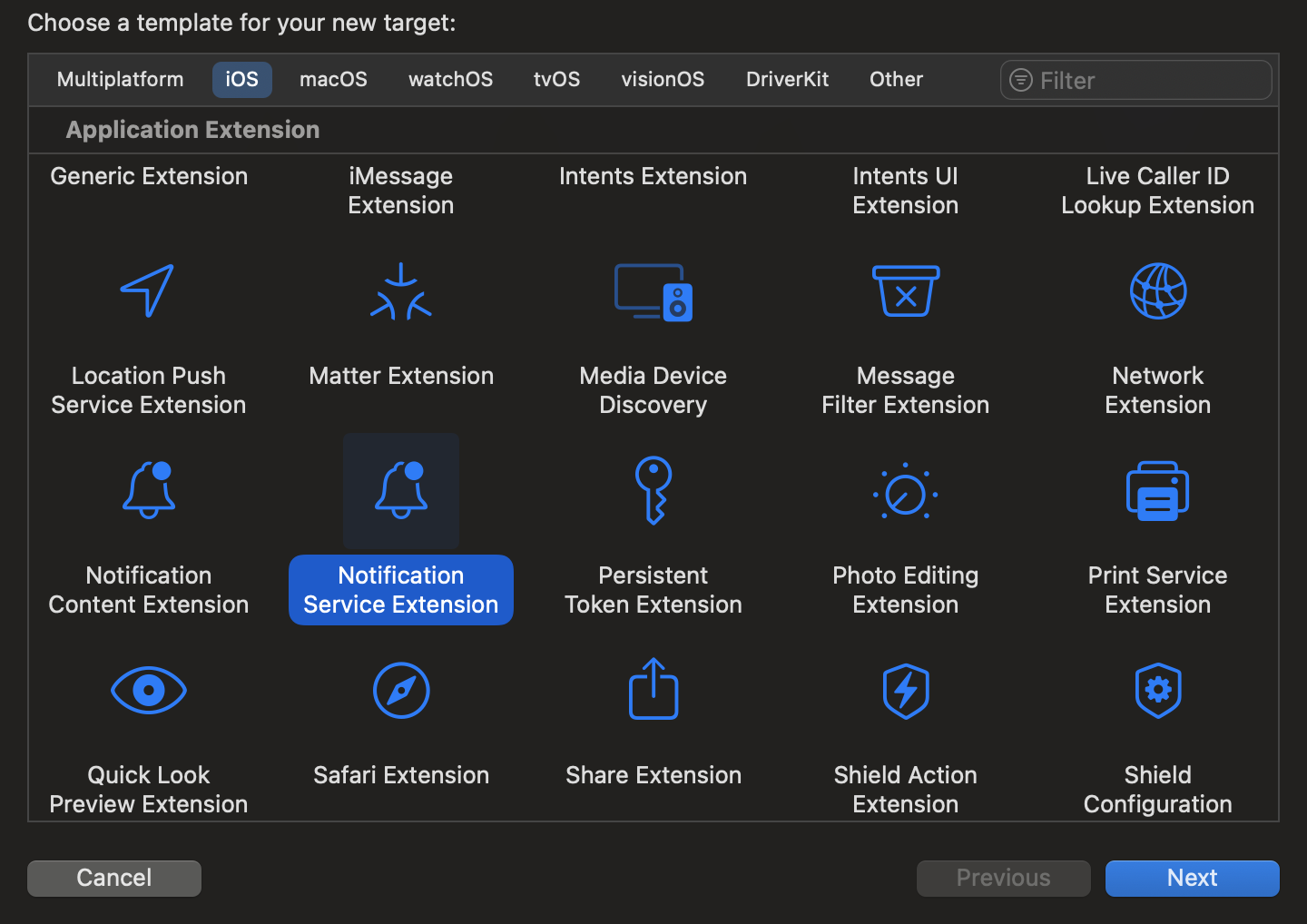
- In the Language list, select Objective-C. Fill out the remaining settings as appropriate.
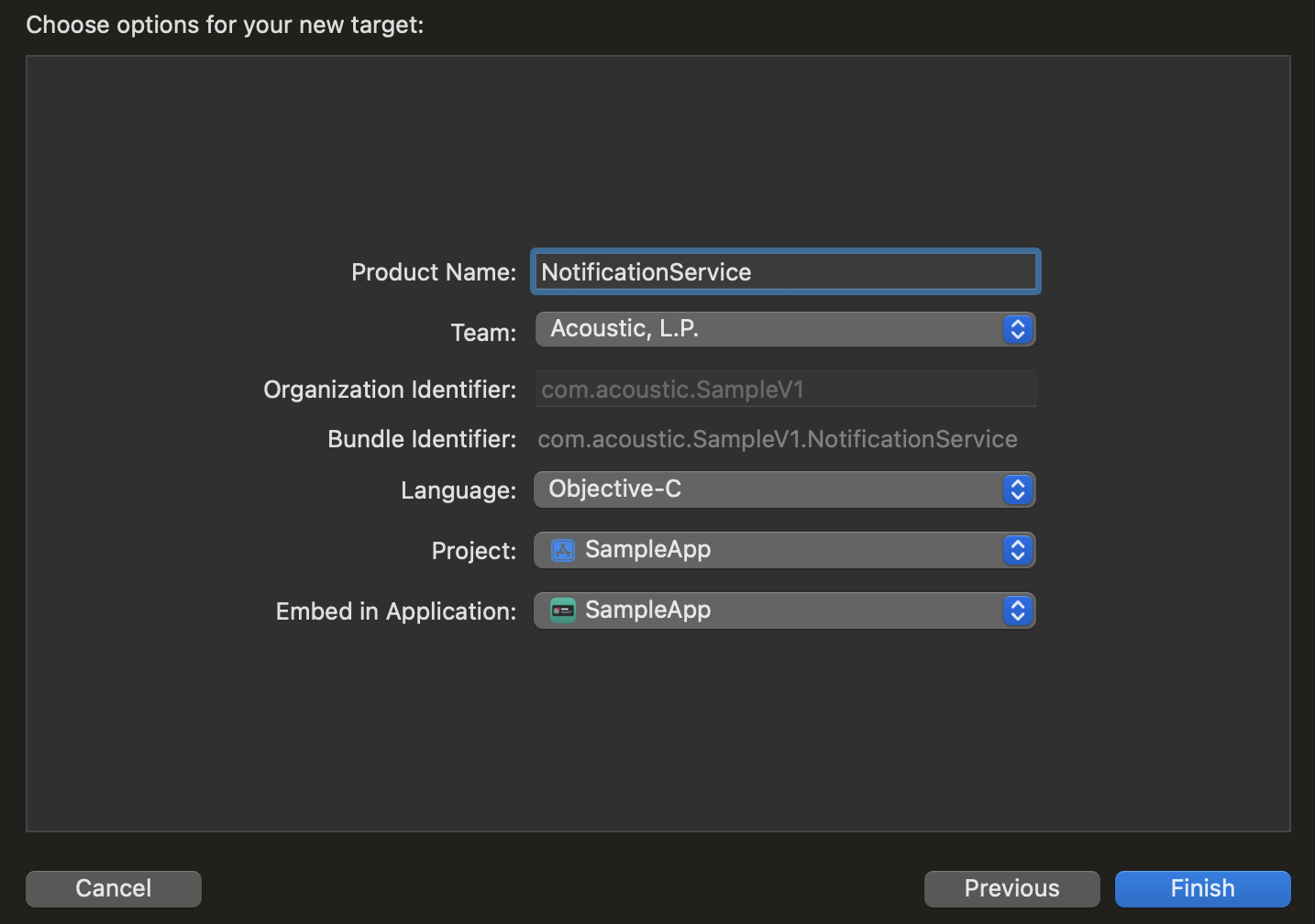
- If a confirmation message appears, select Activate.
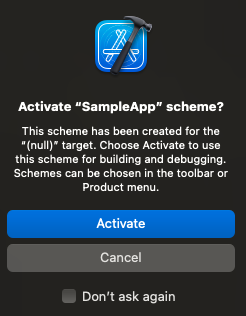
- Find a new target and a new directory in your project.
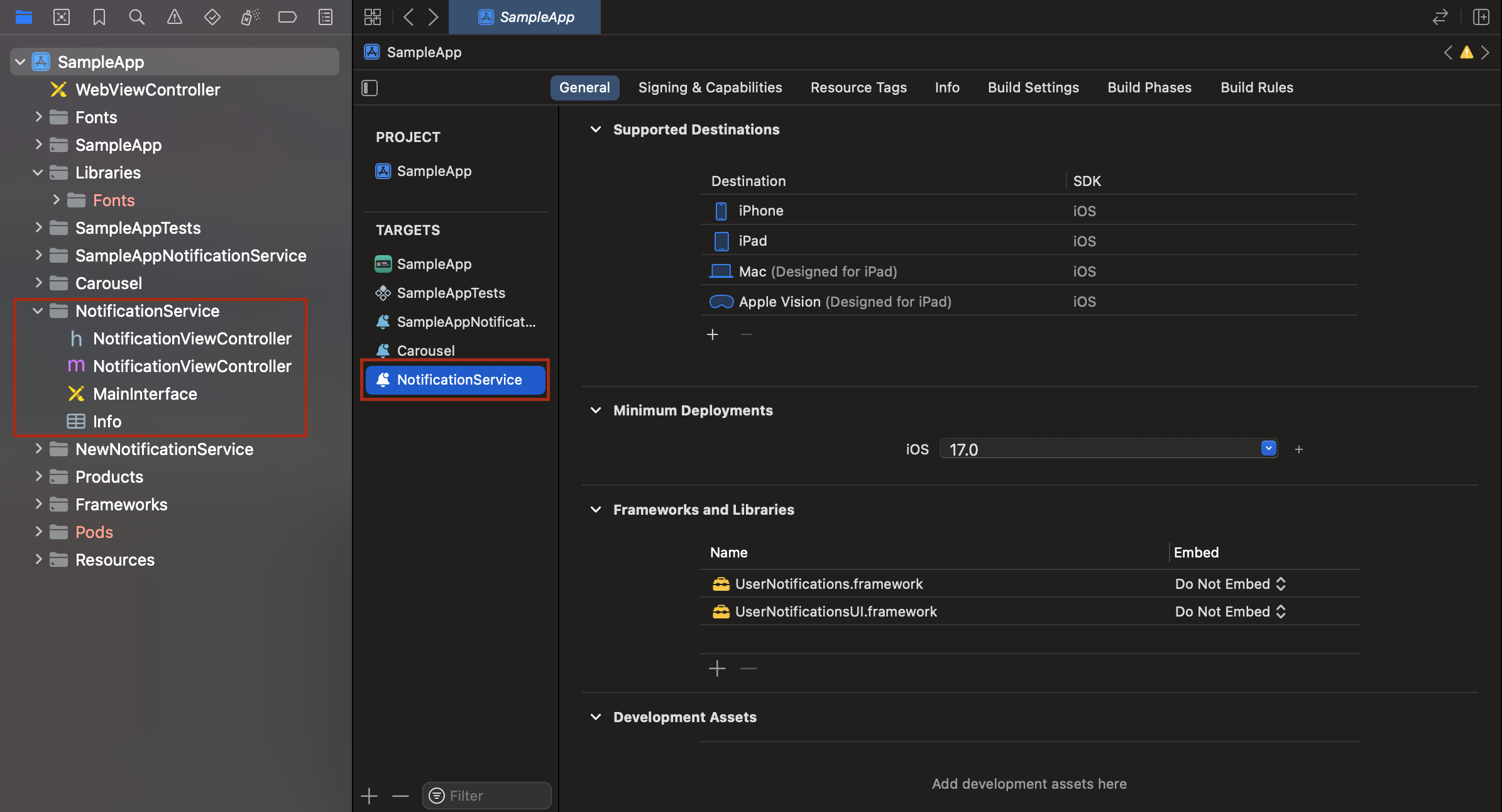
Step B: Configure the Notification Service target
Select the new target and go through the following settings on the General tab.
- Minimum deployments. Make sure the iOS version is the same as in the primary application target.
- Frameworks and Libraries. Add AcousticMobilePushNotification.xcframework and libCordova.a. For AcousticMobilePushNotification.xcframework, the Embed and Sign option must be selected. AcousticMobilePush.xcframework should already be present based on earlier steps in these instructions.
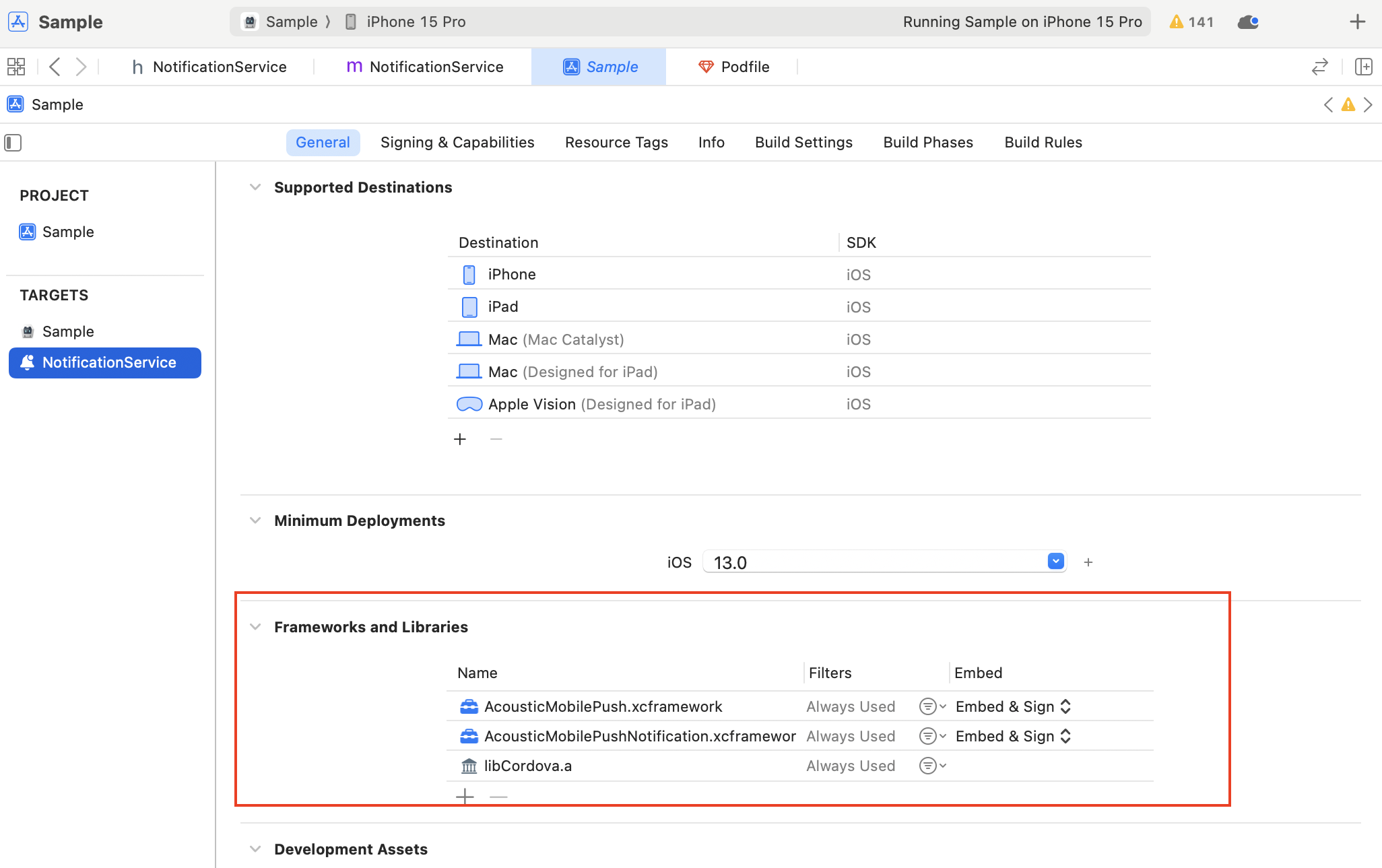
On the Signing & Capabilities tab, add two capabilties: Keychain Sharing and App Groups. Make sure you use the same values as for the primary application target.
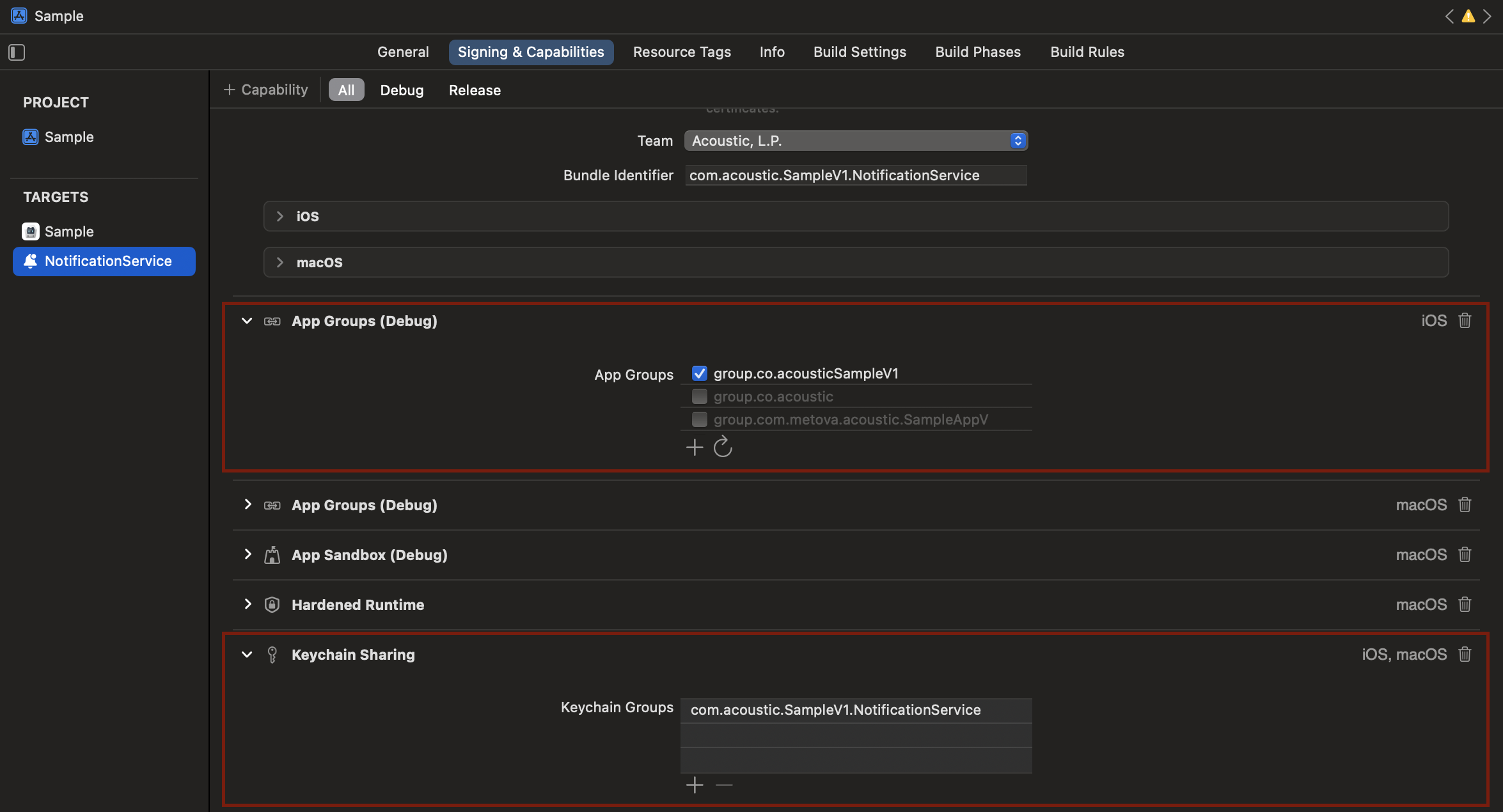
On the Build Settings tab, expand the Linking - General section. Make sure Other Linker Flags is set to -ObjC.
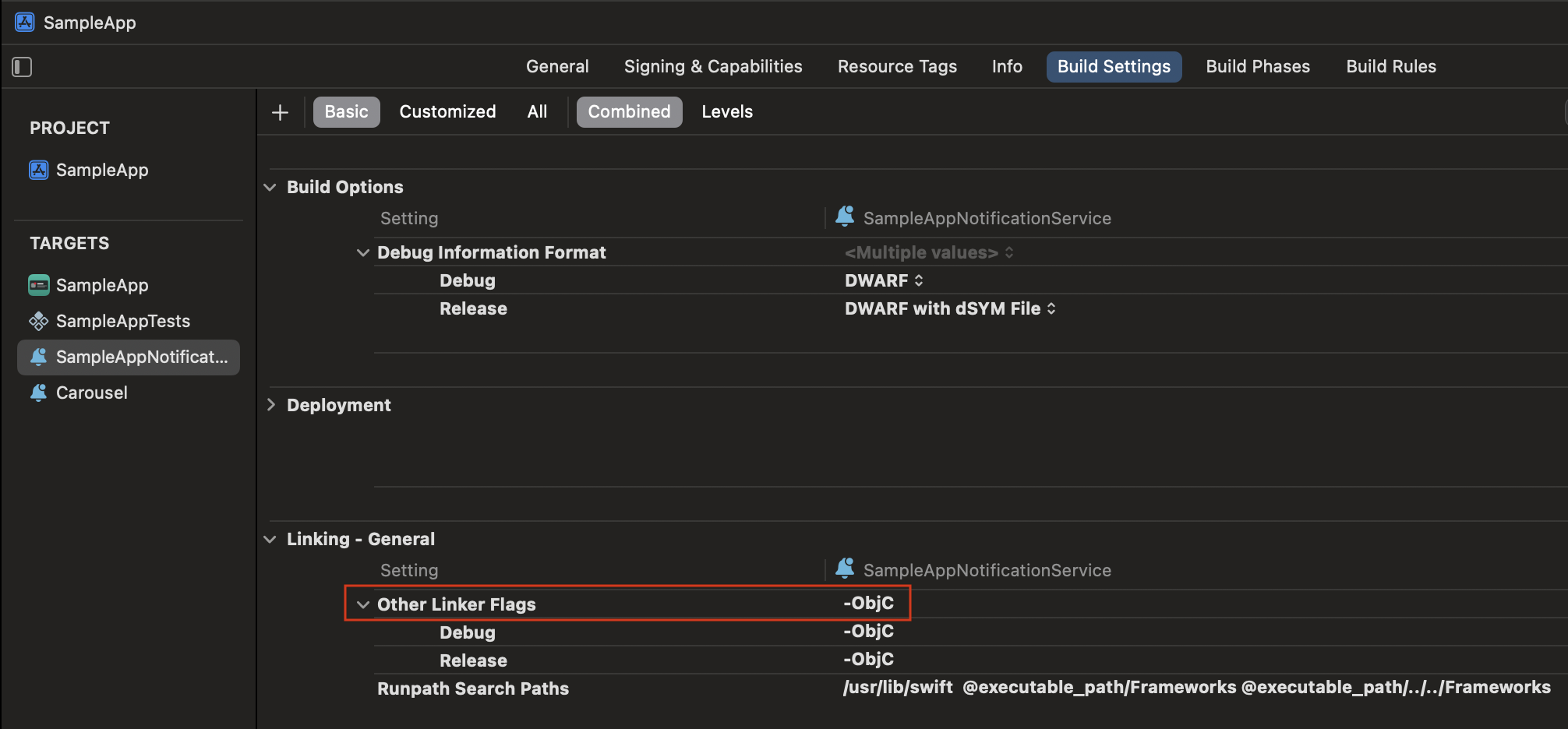
Step C: Update related project files
- In the project navigator, select config.xml. Add the Notification Service target to the file's Target Membership.
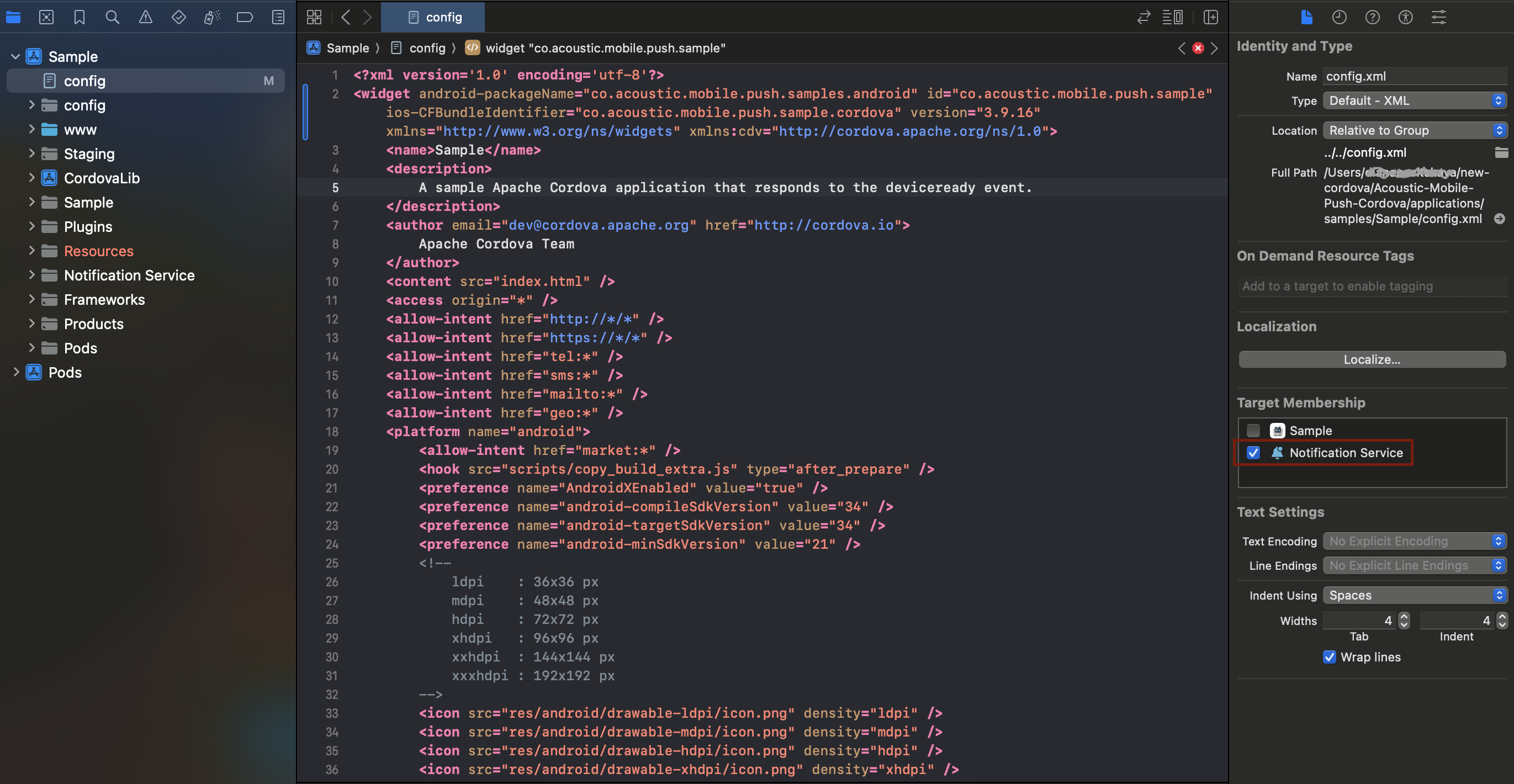
- In the project navigator, open the
Plugins
directory and select MCEManualConfiguration.m. Add the Notification Service target to the file's Target Membership.
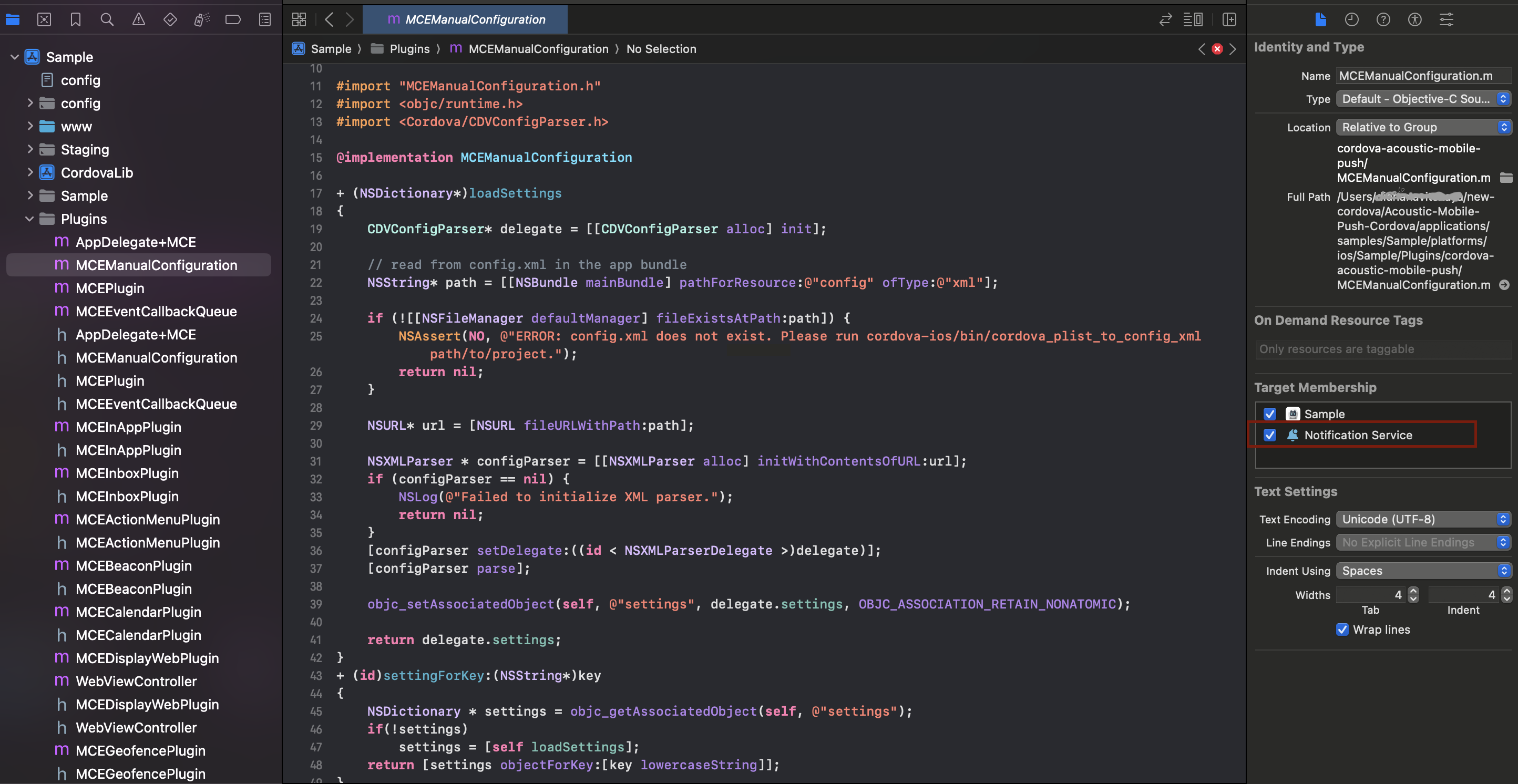
- In the project navigator, open the
Notification Service
directory and select NotificationViewController.h. Import theAcousticMobilePushNotification
framework.
#import <AcousticMobilePushNotification/AcousticMobilePushNotification.h>
- In the same file, add an MCE notification service property.
@property MCENotificationService * notificationService;
- In the same directory, select NotificationViewController.m. Import MCEManualConfiguration.h and AcousticMobilePush.h.
#import "MCEManualConfiguration.h"
#import "AcousticMobilePush/AcousticMobilePush.h"
- Create an initializer method to initialize the
MCENotificationService
property:
- (instancetype)init {
if (self = [super init]) {
[MCEConfig sharedInstanceWithDictionary:MCEManualConfiguration.xmlSettings];
self.notificationService = [[MCENotificationService alloc] init];
}
return self;
}
- Update the
didReceiveNotificationRequest
method to use the notification-action.
if (request.content.userInfo[@"notification-action"]) {
[self.notificationService didReceiveNotificationRequest:request
withContentHandler:contentHandler];
return;
}
- In the
serviceExtensionTimeWillExpire
method, call themce mobile push
method of the same name.
[self.notificationService serviceExtensionTimeWillExpire];
Sample NotificationViewController.h file
@interface NotificationService : UNNotificationServiceExtension
@property MCENotificationService * notificationService;
@end
Sample NotificationViewController.m file
@interface NotificationService ()
@property (nonatomic, strong) void (^contentHandler)
(UNNotificationContent *contentToDeliver);
@property (nonatomic, strong) UNMutableNotificationContent
*bestAttemptContent;
@end
@implementation NotificationService
-(instancetype)init {
if(self = [super init]) {
[MCEConfig sharedInstanceWithDictionary: MCEManualConfiguration.
xmlSettings];
self.notificationService = [[MCENotificationService alloc]
init];
}
return self;
}
- (void)didReceiveNotificationRequest:(UNNotificationRequest *)request
withContentHandler:(void (^)(UNNotificationContent * _Nonnull))
contentHandler {
if(request.content.userInfo[@"notification-action"]) {
[self.notificationService didReceiveNotificationRequest:
request withContentHandler: contentHandler];
return;
}
}
- (void)serviceExtensionTimeWillExpire {
[self.notificationService serviceExtensionTimeWillExpire];
}
@end
Step D: Build and run the app
In a terminal emulator, navigate to the project directory. Now you can build and run your app with the Campaign SDK.
cordova build ios
cordova run ios
- Go to the Firebase Console and download the Firebase Android configuration file (google-services.json). Add the file to the main project directory:
android/app/
. - To apply the changes, run the following Node.js command from the primary project directory.
node node_modules/cordova-acoustic-mobile-push-sdk-beta/scripts/installPlugins.js
- Synchronize your project and run the app.
cordova build android
Updated 8 days ago